I needed a simple way to obtain this simple result: whenever a certain component in the UI (a WebBrowser control) raises some specific event, I want a command in the VM to be executed, without the need to specify any command parameter. The only requirement I want is avoiding a single line of code in the UI :) because, having no code in the UI is one of the main benefit of the MVVM model.
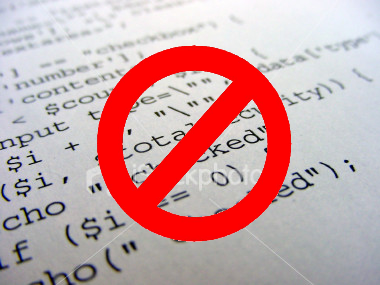
To keep everything simple I want a simple syntax that permits me to specify that when an event of type X is raised, a command of name Y should be called.
1
2
3
4
5
6
7
| <Controls:xxxWebBrowserManagerFlexible x:Name="wbBrowser" Margin="0,0,0,0"
BrowserType="InternetExplorer"
Links="{Binding LinksOfThePage}"
Behaviours:EventToCommandBehavior.Bind="DocumentCompleted-SignalDocumentComplete"
RawHtmlContent="{Binding FullHtmlContent, Mode=TwoWay}"
SelectedText="{Binding BrowserSelectedText, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}"
CurrentUrl="{Binding UrlToNavigate, Mode=TwoWay}" />
|
As you can see I created a simple behavior called EventToCommandBehavior that accepts a very simple syntax: a string of format *EvenName-CommandName.*This solution probably is not so elegant, it has no intellisense or designer support, but it works and it is supersimple. My Behavior has an Attached property called Bind
1
2
3
4
5
6
7
8
| public static readonly DependencyProperty BindProperty =
DependencyProperty.RegisterAttached
(
"Bind",
typeof(String),
typeof(EventToCommandBehavior),
new UIPropertyMetadata(String.Empty, OnBindChanged)
);
|
All the dirty work is done inside the OnBindChanged.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
| private static Dictionary<Object, String> _routes
= new Dictionary<object, String>();
private static void OnBindChanged(DependencyObject dpo, DependencyPropertyChangedEventArgs args)
{
if (String.IsNullOrWhiteSpace((String) args.NewValue)) return;
String[] bind = ((String) args.NewValue).Split('-');
EventInfo ev = dpo.GetType().GetEvent(bind[0]);
MethodInfo handler = typeof(EventToCommandBehavior)
.GetMethod("Handler", BindingFlags.NonPublic | BindingFlags.Static);
var eh = Delegate.CreateDelegate(ev.EventHandlerType, null, handler);
var minfo = ev.GetAddMethod();
minfo.Invoke(dpo, new object[] { eh });
//now go for the command.
_routes.Add(dpo, bind[1]);
}
|
The core part is finding the EventInfo for choosen event, and create dynamically an handler capable to handle every event. The association between EventName and CommandName is stored inside a dictionary object, and the handler is really simple, just get a reference to the command with reflection and call the Execute method.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
| private static void Handler(Object sender, EventArgs e)
{
String commandName;
if (_routes.TryGetValue(sender, out commandName))
{
FrameworkElement fe = sender as FrameworkElement;
if (fe.DataContext != null)
{
PropertyInfo pinfo = fe.DataContext.GetType().GetProperty(commandName);
if (pinfo != null)
{
ICommand command = (ICommand) pinfo.GetValue(fe.DataContext, null);
if (command.CanExecute(null))
{
command.Execute(null);
}
}
}
}
}
|
Since the View Model that contains the ICommand is the DataContext of the element that raised the event, I simply use the GetProperty() method to find the right ICommand, and finally call the Execute method to actually call the command. Since one of the prerequisites states that we need to pass no command parameters, I simply pass null to Execute method.
Alk.